Pythonでディレクトリを選択するダイアログを使う
Pythonでディレクトリを選択するダイアログを表示して使ってみます。
ディレクトリを選択する
いわゆるコモンダイアログでディレクトリを選択します。
Pythonのtkinterを使います。
import tkinter.filedialog
ret = tkinter.filedialog.askdirectory([initialdir], [title], [mustexist])
変数 |
型 |
内容 |
---|---|---|
initialdir |
str |
省略可。ダイアログを開いた時点の初期ディレクトリ。 |
title |
str |
省略可。ダイアログのタイトル。 |
mustexist |
bool |
省略可。既定値はTrue。存在するディレクトリだけを選択できるようにするか。 |
ret |
str |
ディレクトリの絶対パス。 |
mustexistで存在しないディレクトリを選択できるかどうかを指定します。mustexistがTrueの場合は、既存のディレクトリだけを選択できます。mustexistがFalseの場合は、入力欄に新しいディレクトリ名を入れて選択することが出来ます。
試してみましょう。
ボタンを押すとディレクトリを選択するダイアログを表示して、ダイアログを閉じると戻り値の型と内容をラベルに表示するアプリです。
import tkinter
import tkinter.filedialog
class Application(tkinter.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.master.title('tkinter dialog trial')
self.pack()
self.create_widgets()
def create_widgets(self):
self.dialog_button = tkinter.Button(self, text='Choose Directory...', command=file_open, width=120)
self.dialog_button.pack(anchor=tkinter.NW)
self.text_1 = tkinter.StringVar()
self.type_label = tkinter.Label(self, textvariable=self.text_1)
self.type_label.pack(anchor=tkinter.W)
self.text_2 = tkinter.StringVar()
self.content_label = tkinter.Label(self, textvariable=self.text_2)
self.content_label.pack(anchor=tkinter.W)
def file_open():
ini_dir = 'C:\\Program Files\\Python37'
ret = tkinter.filedialog.askdirectory(initialdir=ini_dir, title='file dialog test', mustexist = True)
app.text_1.set('Type : ' + str(type(ret)))
app.text_2.set('Content : ' + str(ret))
root = tkinter.Tk()
app = Application(master=root)
app.mainloop()
まずはmustexistがTrueの場合です。
ダイアログでtoolsディレクトリを選択します。
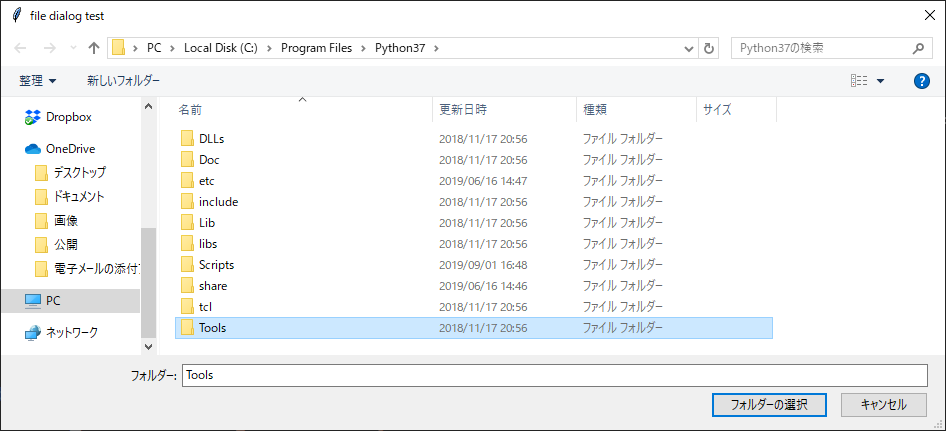

ディレクトリのパスが文字列として戻されました。
ではここで、存在しないディレクトリを選択してみます。
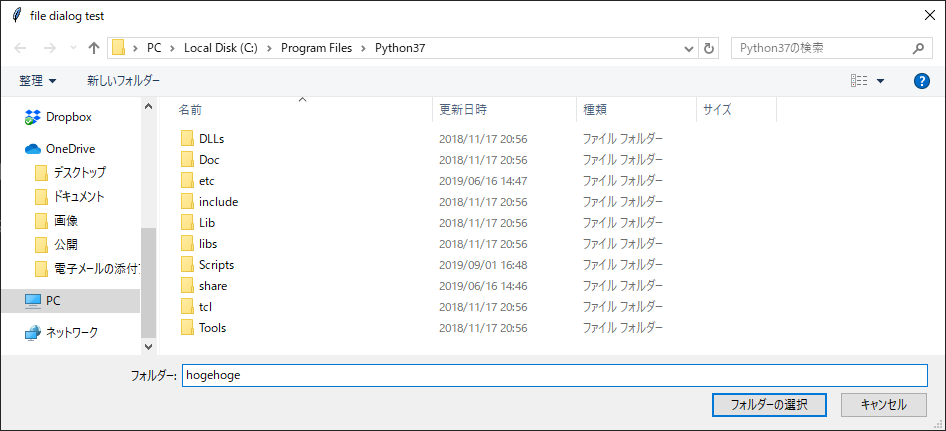
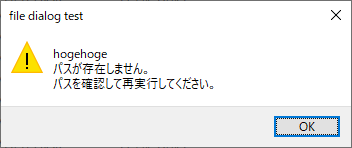
ディレクトリが存在しないと怒られてしまいました。ダイアログに戻ります。
では、mustexistをFalseにしてみます。
def file_open():
ini_dir = 'C:\\Program Files\\Python37'
ret = tkinter.filedialog.askdirectory(initialdir=ini_dir, title='file dialog test', mustexist=False)
app.text_1.set('Type : ' + str(type(ret)))
app.text_2.set('Content : ' + str(ret))
これで存在しないディレクトリが選択できるはず・・・なのですが、なぜか私の環境(Windows10、Python 3.7)ではうまくうごきませんでした。
まあダイアログ内で右クリックメニューが使えますので、ディレクトリを作ってから選択すれば良いのかもしれませんが。
ちなみに、ダイアログをキャンセルすると、空の文字列が戻されます。
公開日
広告
PythonでGUIカテゴリの投稿
- PythonからWindowsのクリップボードに画像をコピーする
- PythonでCanvasをリサイズできるようにしてみた
- PythonでGUIに画像を表示する
- Pythonでクリップボードとのデータのやりとりをする
- Pythonでグラフ(Matplotlib)を表示して動的に変更する
- Pythonでディレクトリを選択するダイアログを使う
- Pythonでファイルを開くダイアログを使う
- Pythonで名前を付けて保存するダイアログを使う
- PythonのGUIを試してみた
- Pythonのcanvasにマウスで線を描いてみる
- Pythonのcanvasに表示した四角形を変形する
- Pythonのcanvasのマウスポインタの座標を取得する
- Pythonのtkinterのcanvasにクリップボードから画像をペーストする
- Pythonのtkinterのcanvasに表示する画像を切り替える